Start / Stop SharePoint Services via PowerShell
If you have installed SharePoint on your workstation, you might want to start the services only in case of active development. Otherwise the services consume memory and other resources.
The PowerShell script in this post will allow you to easily start and stop all required services for a local SharePoint Installation.
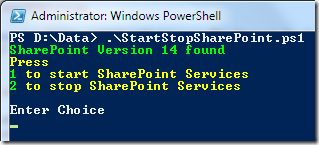
It will detect if you’ve installed SharePoint 2007/WSS, SharePoint 2010 or SharePoint 2013. Just copy the code below to a file, name it StartStopSharePoint.ps1 (yes, very creative :-)) and call it from a PowerShell (you don’t need the SharePoint Management PowerShell).
$SharePointV3Services = ('SQLSERVERAGENT','MSSQLSERVER','W3SVC','SPWriter','SPTrace','SPTimerV3','SPAdmin','OSearch','DCLoadBalancer','DCLauncher') $SharePointV4Services = ('SQLSERVERAGENT','MSSQLSERVER','W3SVC','SPSearch4','OSearch14','SPWriterV4','SPUserCodeV4','SPTraceV4','SPTimerV4','SPAdminV4','FIMSynchronizationService','FIMService','DCLoadBalancer14','DCLauncher14') $SharePointV15Services = ('SQLSERVERAGENT','MSSQLSERVER','W3SVC','SPSearchHostController','OSearch15','SPWriterV4','SPUserCodeV4','SPTraceV4','SPTimerV4','SPAdminV4','FIMSynchronizationService','FIMService','DCLoadBalancer15','DCLauncher15') $Services = $SharePointV3Services $version = 12 # check for SharePoint 2010 $hive = get-ItemProperty "hklm:\SOFTWARE\Microsoft\Shared Tools\Web Server Extensions\14.0" if ($hive -eq $null) { if ((get-ItemProperty "hklm:\SOFTWARE\Microsoft\Shared Tools\Web Server Extensions\15.0" SharePoint).SharePoint -eq 'Installed') { $version = 15 $Services = $SharePointV15Services } } else { if ((get-ItemProperty "hklm:\SOFTWARE\Microsoft\Shared Tools\Web Server Extensions\14.0" SharePoint).SharePoint -eq 'Installed') { $version = 14 $Services = $SharePointV4Services } } Write-Host -ForegroundColor Green 'SharePoint Version' $version 'found' Write-Host -ForegroundColor Yellow 'Press' Write-Host -ForegroundColor Green '1' -NoNewline Write-Host -ForegroundColor Yellow ' to start SharePoint Services' Write-Host -ForegroundColor Green '2' -NoNewline Write-Host -ForegroundColor Yellow ' to stop SharePoint Services' Write '' function StartSharePoint($Services) { Write-Host -ForegroundColor Yellow ('Starting ' + $Services.Length + ' services') foreach ($serviceName in $Services) { $service = Get-Service $serviceName -ErrorAction SilentlyContinue if ($service) { $startMode = (get-wmiobject win32_service | where-object {$_.Name -eq $serviceName}).StartMode if (($service.Status -ne 'Running') -and ($startMode -ne 'Disabled')) { Write-Host -ForegroundColor Green ('Starting "' + $service.DisplayName + '"') Start-Service $serviceName } } else { Write-Host -ForegroundColor Red ('Service "' + $serviceName + '" does not exist') } } } function StopSharePoint($Services) { Write-Host -ForegroundColor Yellow ('Stopping ' + $Services.Length + ' services') for($i = ($Services.Length - 1); $i -gt -1; $i--) { $serviceName = $Services[$i] $service = Get-Service $serviceName -ErrorAction SilentlyContinue if ($service) { $startMode = (get-wmiobject win32_service | where-object {$_.Name -eq $serviceName}).StartMode if ($service.Status -ne 'Stopped') { Write-Host -ForegroundColor Green ('Stopping "' + $service.DisplayName +'"') Stop-Service $serviceName -force } else { Write-Host -ForegroundColor Green ('"' + $service.DisplayName + '" is already stopped') } } else { Write-Host -ForegroundColor Red ('Service "' + $serviceName + '" does not exist') } } } function UserInput($strMsg, $intStyle) { #$strMsg: Message displayed on the console sreen #$intStyle: User Input Style: #1 = Read-Host (Multiple characters, require to press Enter); #2 = $host.ui.rawui.readkey() (Single character, do not require to press enter) switch($intStyle) { 1 { $strInput = Read-Host $strMsg ; $host.ui.rawui.foregroundcolor = $Global:fgColor ; return $strInput } 2 { Write-Host $strMsg; $strInput = $host.UI.RawUI.ReadKey("NoEcho,IncludeKeyDown"); return $strInput} } } $userInput = UserInput "Enter Choice" 2 switch ($userInput.VirtualKeyCode) { 49 { StartSharePoint($Services) } 50 { StopSharePoint($Services) } default { Write-Host -ForegroundColor Red 'Press "1" or "2"' } }
Hint: In case you haven’t set the Script-Execution-Policy yet, you will be prompted to do so. –> Using the Set-ExecutionPolicy Cmdlet
Happy Coding!